0%
Kiraray
Optix Shader
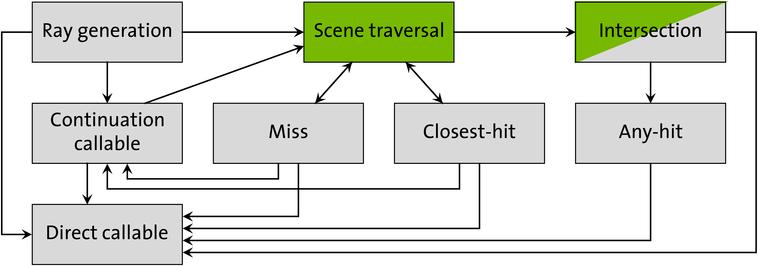
- Ray generation
- Intersection
- Any-hit
- Called when a traced ray finds a new, potentially closest,
intersection point, such as for shadow computation. See “Ray
information”
- 每次 intersection 都会被调用
- Closest-hit
- Miss
- Exception
- Exception handler, invoked for conditions such as stack overflow and
other errors. See “Exceptions”.
- Direct callables
- Similar to a regular CUDA function call, direct callables are called
immediately. See “Callables”.
- Continuation callables
- Unlike direct callables, continuation callables are executed by the
scheduler. See “Callables”.
MegaKernel
- 一根光线的所有过程都写在 raygen 中
- divergence:不同像素的光线的期望长度可能不一致
RadianceRay
- raygen
- NEE:击中场景中点的时候,对这个点做 NEE
- NEE 实现的时候,可以采样环境光,因此也需要和环境光做 MIS
- 不 NEE 的情况
- 材质为镜面(specular)
- 直接光照(第一跳,depth=0)
- 采样光源
- 简单实现:先采样一个光源(\(\dfrac{1}{N}\)),再在光源上均匀采样
- 如果采样的光源位置被另外一个光源遮挡了,那么 NEE 部分的贡献为 0
- 实现简单,不需要考虑某一个方向能够被多个光源采样到的情况(远处的直接被剔除了)
- 面积采样要转化为立体角采样,推导
- 代码:
src/core/shape.h:sample()
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42
| _ raygen() { for(spp) { tracePath(); } }
_ tracePath() { for(depth) { optixTrace(); if(miss){ handleMiss(); break; }
if(light) { handleHit(); }
if(shouldStop) { break; }
throughput /= RR;
if(NEE) { }
if(genScatterRay()) { break; } } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| _ ahit() { }
_ chit() { }
_ miss() { }
|
ShadowRay
- shadow ray 在 NEE 的时候使用,判定光线能否打到光源
1 2 3 4
| trace_flags = OPTIX_RAY_FLAG_TERMINATE_ON_FIRST_HIT | OPTIX_RAY_FLAG_DISABLE_CLOSESTHIT;
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| _ ahit() { }
_ chit() { }
_ miss() { }
|
WaveFront
- 注意:PT 部分 item 都是
soa
的
- 场景构建中:
soa
部分,将 array of struct
转化为 struct of array
- https://github.com/crosetto/SoAvsAoS
- 提供多线程访问的时候的缓存一致性
- 简写
1 2 3 4 5 6 7
| RayQueue* rayQueue[2]{ }; MissRayQueue* missRayQueue{ }; HitLightRayQueue* hitLightRayQueue{ }; ShadowRayQueue* shadowRayQueue{ }; ScatterRayQueue* scatterRayQueue{ }; PixelStateBuffer* pixelState;
|
- 流程
- 只有在使用到 optix 函数的时候再使用
optixLaunch()
,其他的直接调用 cuda kernel 并行实现
- 使用
optixLaunch()
的函数:trace_closest()
、trace_shadow()
- 优化:如果没有环境光,那么就不需要 miss queue
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| for(spp) { reset_current_ray_queue(); generate_camera_rays();
for(depth) { prepara_for_current_depth(); trace_closest();
handle_hit(); handle_miss();
if(depth == MaxDepth) { break; }
();
if (NEE) { trace_shadow(); } }
gather(); }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| _ raygen_closest() { optixTrace(); }
_ chit_closest() { prepareShadingData();
push_into_corresponding_queue(); }
_ ahit_closest() { }
_ miss_closest() { }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| _ raygen_shadow() { optixTrace(); if(miss) { } }
_ chit_shadow() { }
_ ahit_shadow() { }
_ miss_shadow() { }
|