0%
Learn Git
基础
确认修改 commit
分支 branch
合并分支 merge
- 将
branch1
分支合并到当前分支
branch1
中的头指针没有变换,当前分支的头指针指向合并后的节点
例子
1 2 3
| A---B---C topic / D---E---F---G master*
|
1 2 3
| A---B---C topic / \ D---E---F---G---H master*
|
合并分支 rebase
- 将当前分支的修改在
branch1
中都执行一遍,然后当前分支的头指针指向修改后的节点
1
| git rebase branch1 nowBranch
|
- Q:如果说没有删除当前分支头指针指向的原来的那个节点,如何回到这个节点呢?
- 实际上的 git 会删除原来的节点(删除没有引用的节点)
rebase
的含义:将当前分支和 branch1
的分支节点往后移动到指定的节点
例子
1 2 3
| A---B---C topic* / D---E---F---G master
|
1 2 3
| B'---C' topic* / D---E---F---G master
|
rebase/merge
rebase
让提交树更干净(一条线),但是会修改提交历史
merge
不会修改提交历史,但是提交树更复杂
删除分支
1
| git branch --delete branch_name
|
- 注意不能删除当前分支(需要先
checkout
到其他分支)
高级

Description
栏下,蓝色的小圆圈就是
head
的位置
分离头指针
1 2 3 4 5
| cat ./.git/HEAD
type .\.git\HEAD
|
1 2
| git checkout main git checkout hashcode
|
相对引用
1 2 3
| git checkout 092d58b38f41f9594c0c284ef6defc6aba788e8e
git checkout 092d5
|
^
^
表示父节点
- 例如移动
head
到 flower
的父节点
- 加数字表示移动到第几个父提交(存在多个父节点的时候)
加数字
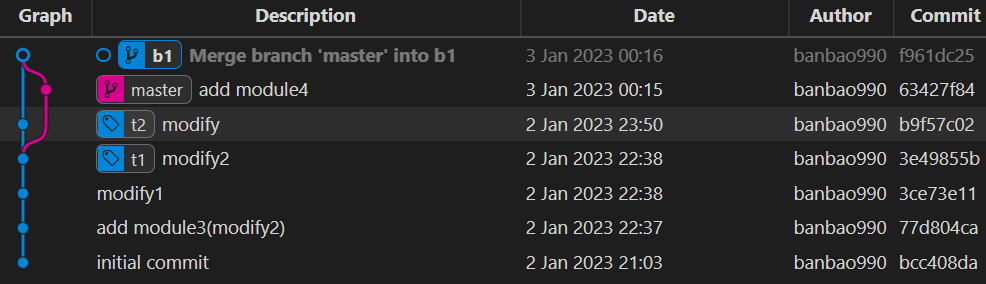
1 2
| git checkout HEAD^^ git checkout HEAD^^1
|
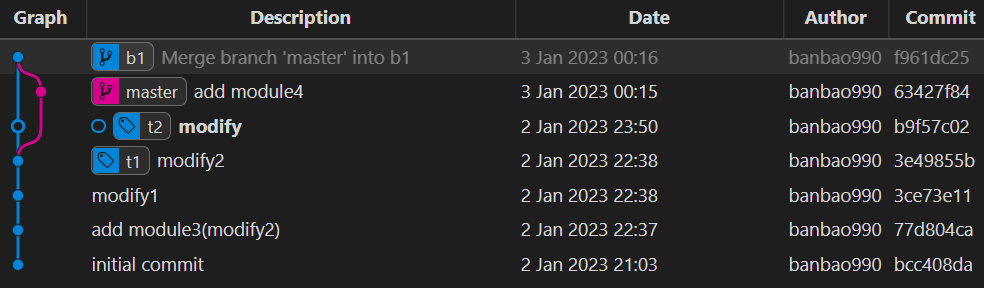
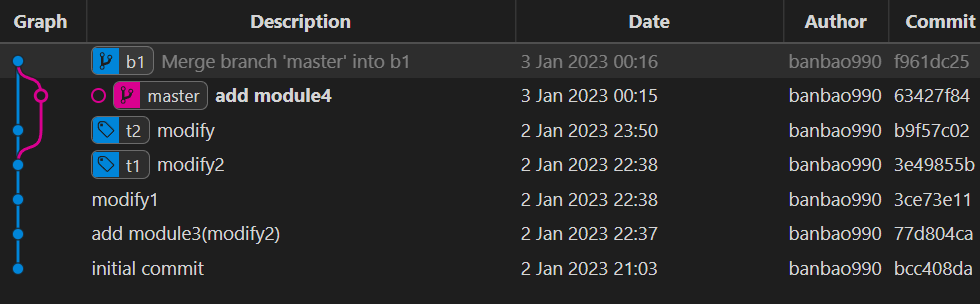
~
链式连接
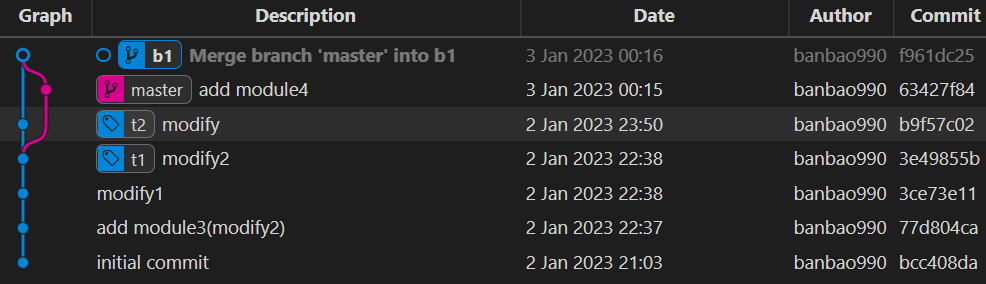
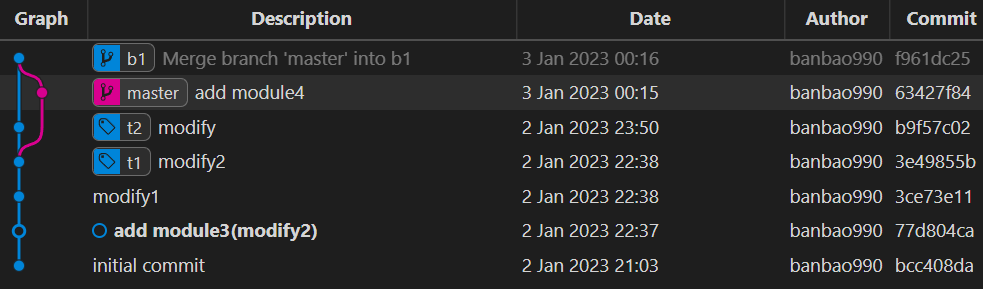
强制修改分支位置
- 将
main
分支强制指向 HEAD
的第
3
级父提交
1
| git branch -f main HEAD^3
|
撤销变更

reset
- 用于本地分支的修改
- 相当于把哈希值为
e816**
结点之后的 commit
都取消了
image-20230102154651492
revert
- 远程分支修改,用于合作的同步
- 新增加一个节点,这个节点便是取消
f9f7**
之后的修改
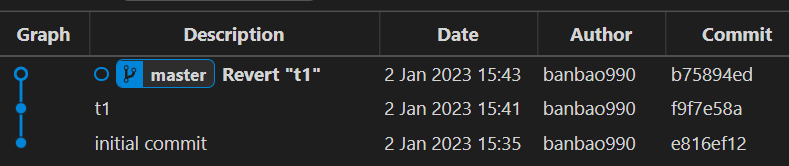
另外的细微区别
git reset hashcode
:表示恢复到 hashcode
节点的状态
- 例如在上面的原始状态,运行
git reset f9f7
,则无事发生(因为当前状态就是
f9f7**
的状态)
git reset hashcode
:表示撤销 hashcode
节点之后(包括这个节点)的修改
- 例如在上面的原始状态,运行
git reset f9f7
,则回到前一个结点 e816**
的状态
移动提交记录
cherry-pick
- 将某个节点上的 commit 在当前节点上进行 commit
- 可以接多个修改
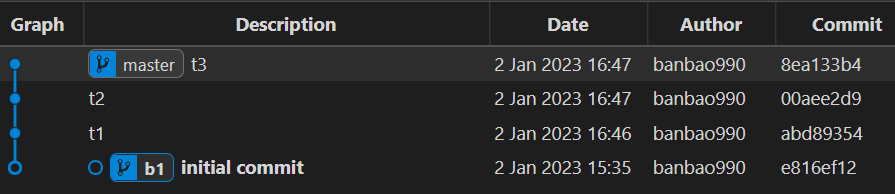
1
| git cherry-pick abd8 00ae 8ea1
|
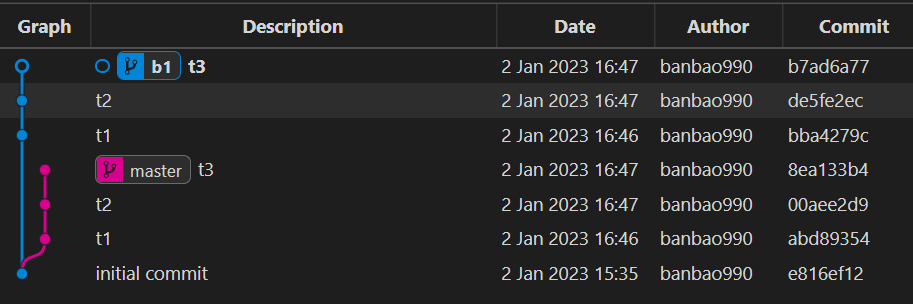
1
| (abd8^, 8ea1] = [abd8, 8ea1]
|
1 2 3 4 5
| git cherry-pick abd8^..8ea1
git cherry-pick abd8^^..8ea1
|
交互式 rebase
1 2
| git rebase -i branch1 git rebase --interactive branch1
|
- 可以选择需要当前分支上的哪些修改
- 可以选择
pick/omit
(还有更多选项)
- 可以修改 commit 的顺序
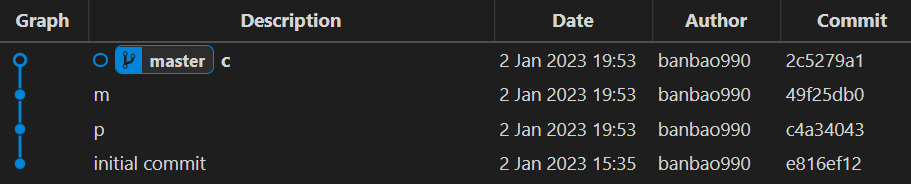
1 2 3 4
| # git-rebase-todo pick 2c5279a c drop c4a3404 p pick 49f25db m
|

应用
debug
- debug 的时候,某些 commit 适用于输出调试信息的,debug
结束之后可以不需要这些调试的commit
1 2 3 4
| main / A--------B--------C--------D bugFix* (debug) (printf) (fixed)
|
1 2
| git checkout main git cherry-pick D
|
1 2 3 4
| D'---main* / A--------B--------C--------D bugFix (debug) (printf) (fixed)
|
修改之前的记录
rebase
image-20230102223848364
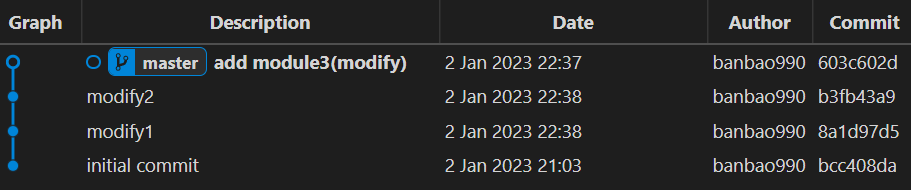
1 2
| git add module3.txt git commit --amend
|
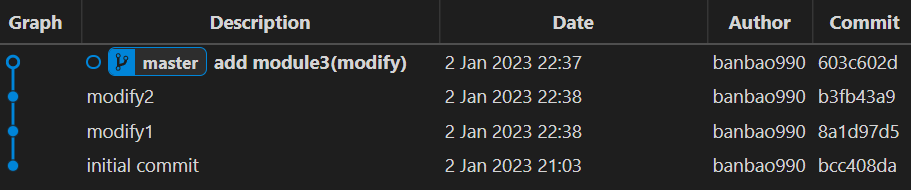
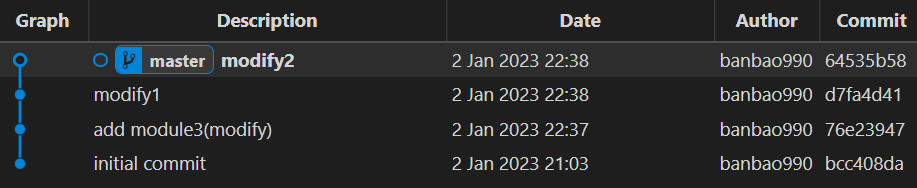
- 实际操作会存在很多冲突,只有在需要修改的模块很独立,提交也比较独立的时候比较方便
cherry-pick
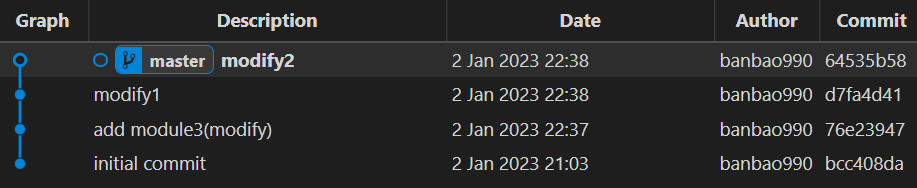
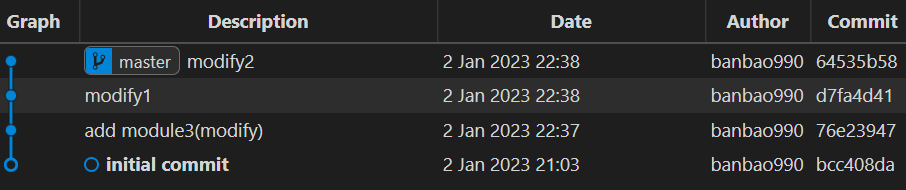
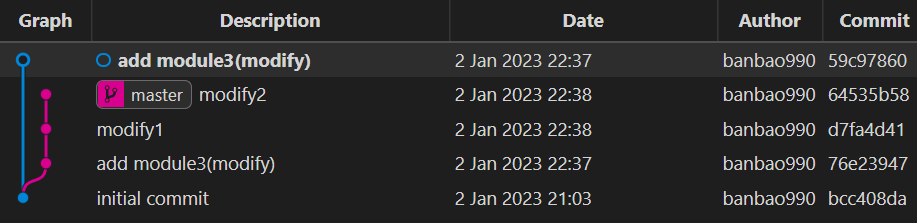
1 2
| git add module3.txt git commit --amend
|
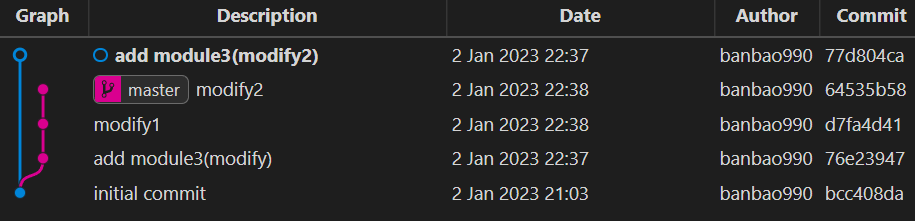
1
| git cherry-pick d7fa^^..6453
|
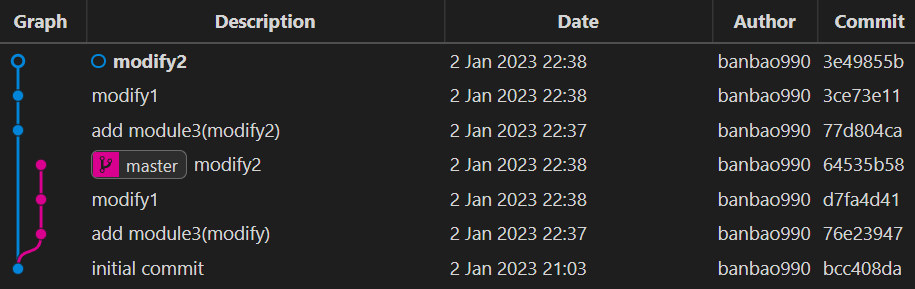
1
| git branch -f master 3e49
|

杂项
tag
- 给节点
Node
打上 tagName
- 可以被作为节点的引用
- 长久留存
1
| git tag -a tagName -m tagMessage
|
describe
- 找到距离节点
hashcode
最近的一个
annotated tag
- 找到距离节点
hashcode
最近的一个 tag
(包括
unannotated tag
)
1
| git describe hashcode --tags
|
1 2 3
| A: tagName B: commit numbers from hashnode C: hashcode's hash number
|
远程
clone
1 2
| git clone git@github.com:github/pycon2011.git git clone https://github.com/github/pycon2011.git
|
- 此时会至少有 3 个分支
master
remotes/origin/HEAD
remotes/origin/master
- 远程分支命名规范:
name/branch
- 切换远程分支
1 2
| git checkout remotes/origin/master git checkout origin/master
|
- 切换远程分支之后
commit
,会形成本地的分离
HEAD
状态
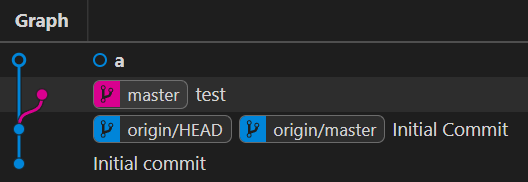
1
| git clone -b branchName remote-link
|
fetch
- 拉取所有远程分支
- 不会更新本地其他分支的状态
- 不改变
HEAD
指针位置
- 合并分支:使用
merge/cherry-pick/merge
等方式
pull
fetch+merge
- 拉取远程分支并合并
- 修改
HEAD
指针位置为最新
push
- 推送本地修改到远程
- 如果是然后会更新本地对应的远程分支状态
push
命令执行之前,会先将本地的记录和远程的同步
- 也就是说如果
push
命令执行失败,需要先
fetch
下来处理冲突之后再 push
1 2 3 4
| git pull --rebase
git fetch git rebase
|
锁定的 master 分支
- 不允许直接推送到远程的主分支,需要推送到其他分支
- 用于权限管理,大型项目开发
- 最好将
master
分支和 remotes/origin/master
分支保持一致
管理
远程追踪
- 当前分支为
master
,本地对应的远程分支为
origin/master
- push
- 推送本地分支到远程
- 更新
origin/master
- pull
- 拉取远程分支,更新
origin/master
- 更新
master
- 默认关联:
master
对应 origin/master
- 自己设定(如下两种方式都 OK)
1 2 3 4 5
| git checkout -b notMasterBranch origin/master
git branch -u origin/master notMasterBranch
|
push 参数
- 在远程仓库
remote
中找到分支
branch
,并将其更新,更新之后更新本地的
origin/branch
- 默认没有参数的时候,
branch
为当前 checkout
的点,此时只有检出点跟踪了某个远程分支,命令才生效
1
| git push remote branch1:branch2
|
- 把本地的
branch1
分支推送到 remote
的
branch2
分支
- 支持相对引用的节点
- 如果
branch2
不存在,会自动新建
fetch 参数
1 2 3 4 5 6 7 8 9
| git fetch remote branch
git fetch remote branch1:branch2
git fetch
|
source 留空
- 神奇操作
- push:远程删除分支
- fetch:本地新建空分支
1 2 3 4 5
| git push origin :side
git fetch origin :bugFix
|
pull
1 2 3 4
| git pull origin foo
git fetch origin foo git merge o/foo
|
1 2 3 4
| git pull origin bar~1:bugFix
git fetch origin bar~1:bugFix git merge bugFix
|
其他
升级 git 版本
1
| git update-git-for-windows
|
git 老是卡死