0%
文件与流
1. IO及流
System.IO
流的分类
- Stream 类
- 按存取位置分
- FileStream、MemoryStream、BufferedStream
- 读写类
- BinaryReader、BinaryWriter
- TextReader、TextWriter
- StreamReader、StreamWriter
- StringReader、StringWriter
- 示例
1 2
| FileStream fs = new FileStream(@"c:\foo.txt", FileMode.Create); StreamWriter writer = new StreamWriter(fs);
|
System.IO 的常用类
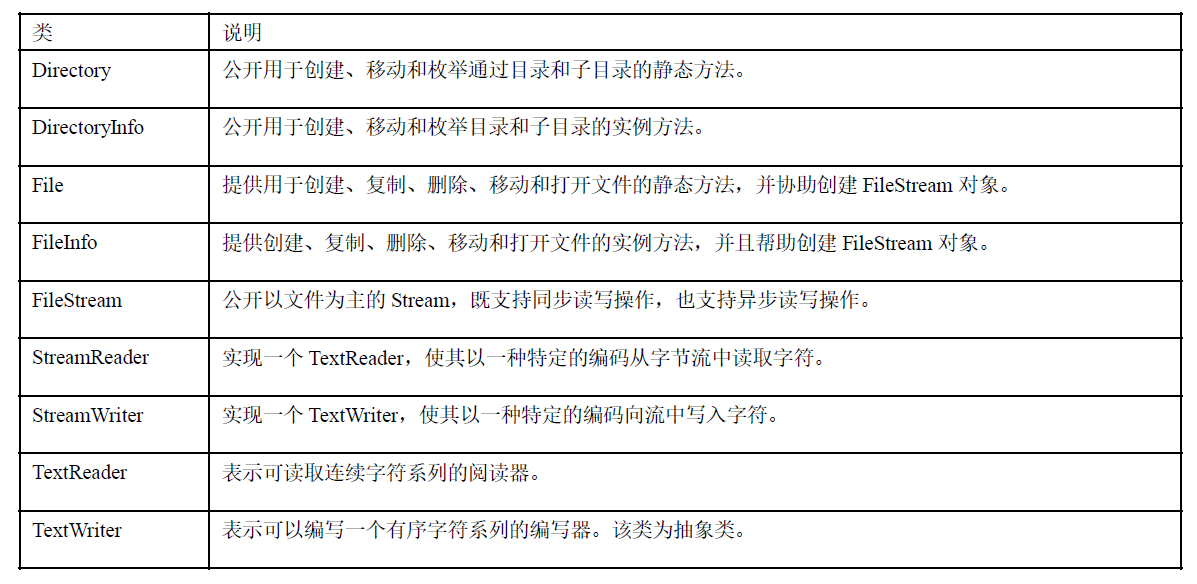
2. 文件及目录
文件和文件夹管理
FileInfo |
具体的文件 |
File |
提供 static 方法 |
DirectoryInfo |
具体的文件夹 |
Directory |
提供 static 方法 |
FileSystemInfo |
FileInfo 及 DirectoryInfo 的父类 |
Path |
文件路径类 |
文件及文件夹的信息
- FileInfo 对象可以获取文件大小等各种信息
- DirectoryInfo 对象可以获取文件夹大小等各种信息
FileInfo
Name |
文件名称 |
Extension |
文件扩展名 |
FullName |
文件完全路径(物理路径) |
Length |
文件大小,单位为字节 |
CreationTime |
文件创建时间 |
LastAccessTime |
文件上次访问时间 |
LastWriteTime |
文件上次修改时间 |
DirectoryName |
所在文件夹 |
Attributes |
文件属性,如只读、隐藏等 |
DirectoryInfo
1
| new DirectoryInfo(文件物理路径);
|
Name |
文件夹名称 |
FullName |
文件夹完全路径(物理路径) |
CreationTime |
文件夹创建时间 |
LastAccessTime |
文件夹上次访问时间 |
LastWriteTime |
文件夹上次修改时间 |
Parent |
父文件夹 |
Root |
所在根目录 |
文件操作
1 2 3 4 5 6
| File.Create(filePath); File.Copy(filePath1,filePath2); File.Move(filePath1,filePath2); File.Delete(filePath); File.Exists(filePath); File.CreateText(filePath);
|
1 2 3 4 5 6
| Directory.CreateDirectory(DirPath); Directory.Move(DirPath1,DirPath2); Directory.Delete(DirPath); Directory.Exists(DirPath); Directory.GetDirectories(DirPath); Directory.GetFiles(DirPath);
|
子文件
- 显示指定文件夹下的子文件夹和子文件
- Directory
- GetDirectories 和 GetFiles的 static 方法
- DirectoryInfo
- GetDirectories 和 GetFiles实例方法
- 这两个方法分别返回 DirectoryInfo 对象数组和 FileInfo 对象数组
Path
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| public static string ChangeExtension(string path, string extension); public static string Combine(string path1, string path2, string path3); public static string Combine(string path1, string path2); public static string Combine(string path1, string path2, string path3, string path4); public static string Combine(params string[] paths); public static string GetDirectoryName(string path); public static string GetExtension(string path); public static string GetFileName(string path); public static string GetFileNameWithoutExtension(string path); public static string GetFullPath(string path); public static char[] GetInvalidFileNameChars(); public static char[] GetInvalidPathChars(); public static string GetPathRoot(string path); public static string GetRandomFileName(); public static string GetTempFileName(); public static string GetTempPath(); public static bool HasExtension(string path); public static bool IsPathRooted(string path);
|
示例:递归查看所有文件
文件监视器
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| using System; using System.IO; public class Watcher { public static void Main() { const string path = @"D:\Code\C_plus_plus\"; FileSystemWatcher watcher = new FileSystemWatcher(); watcher.Path = path; watcher.Filter = "*.cpp"; watcher.NotifyFilter = NotifyFilters.LastAccess | NotifyFilters.LastWrite | NotifyFilters.FileName | NotifyFilters.DirectoryName; watcher.Changed += new FileSystemEventHandler(OnChanged); watcher.Created += new FileSystemEventHandler(OnChanged); watcher.Deleted += new FileSystemEventHandler(OnChanged); watcher.Renamed += new RenamedEventHandler(OnRenamed); watcher.EnableRaisingEvents = true; Console.WriteLine("Press'q' to quit the sample."); while (Console.Read() != 'q') ; } public static void OnChanged(object source, FileSystemEventArgs e) { Console.WriteLine("File: " + e.FullPath + " " + e.ChangeType); } public static void OnRenamed(object source, RenamedEventArgs e) { Console.WriteLine("File: {0} renamed to {1}", e.OldFullPath, e.FullPath); } }
|
3. 文件内容处理
文本文件的操作
- StreamReader、StreamWriter
1 2 3 4 5 6 7 8 9 10 11 12
| new StreamReader("文件路径", FileEncode); ReadLine(); ReadToEnd(); Read(); Peek(); Close();
new StreamWriter("文件路径", FileMode, FileEncode) WriteLine(); Write(); Flush(); Close();
|
二进制文件的操作
1 2 3
| new FileStream("文件路径", FileMode, FileAccess); Seek(); Read();
|
示例代码
1 2 3 4 5 6 7 8 9
| try { string fname = LogFile; StreamWriter writer = new StreamWriter(fname, true, System.Text.Encoding.Default); writer.Close(); } catch { }
|
1 2 3 4 5 6
| FileStream fin = new FileStream(infname, FileMode.Open, FileAccess.Read); FileStream fout = new FileStream(outfname, FileMode.Create, FileAccess.Write);
StreamReader brin = new StreamReader(fin, System.Text.Encoding.Default); StreamWriter brout = new StreamWriter(fout, System.Text.Encoding.Default);
|
序列化与反序列化
4. 注册表操作
什么是注册表
- 存放计算机运行方式的配置信息的树状表
- 其中包括 Windows
操作系统配置信息、应用程序配置信息、专用用户设备配置信息、环境配置信息等
打开注册表
编程相关类
- Registry、RegistryKey
- 编程实例